Table of Contents
A lot of online sellers like WooCommerce because of its simplicity and convenience. Anyone can create a website of their own with a few simple setup steps or install a few plugins.
But not every website brought to users the best experience and an “add to cart” action is an example.
Having to reload the entire website every time a customer adds a product to the cart will limit the user experience. Sometimes, this also limits your opportunity to reach out to potential customers.
That is the reason why you have entered this article. In this, I will show the cause and how to install the “Ajax add to cart” button which makes your website smoother.
What is AJAX?
AJAX stands for Asynchronous Javascript and XML is a method of exchanging data with the server without reloading the existing web page.
It is written in Javascript and runs on the client-side so that every browser runs independently.
And this is done by sending an XMLHttpRequest object containing the new information to a web server, which returns the new information only for the portion that has changed.
How does it work?
From the client browser, we have an event to call Ajax. At that time, JavaScript will create an object XMLHTTPRequest. And this object will be sent a request to the server.
For example, when the user clicks on the Input box and selects the skill to find the user’s job. Then we will take that information and send it to the server and need to return the corresponding actions suitable for users.
When the server receives HTTPRequest it will process the request and return the response to the web.
The server processes JavaScript jobs such as the skill that users need and returns the jobs’ data.
After receiving a response from the server, JavaScript will process and update the website for us.
Benefits of “Ajax add to cart”
The reason why you should choose an “Ajax add to cart” for your website are:
- It only sends the needed data and reloads a small part instead of the whole page to update the information.
- The website created will be smoother.
- It increases users’ experience.
- It’s a highly useful tool for those who want to make their online store experience as user-friendly as possible.
There are 2 ways to set up an “Ajax add to cart” button:
For common users:
It is easier for most users who don’t know how to code that you can download it from here. The plugin is free and it works for both single and variable products.
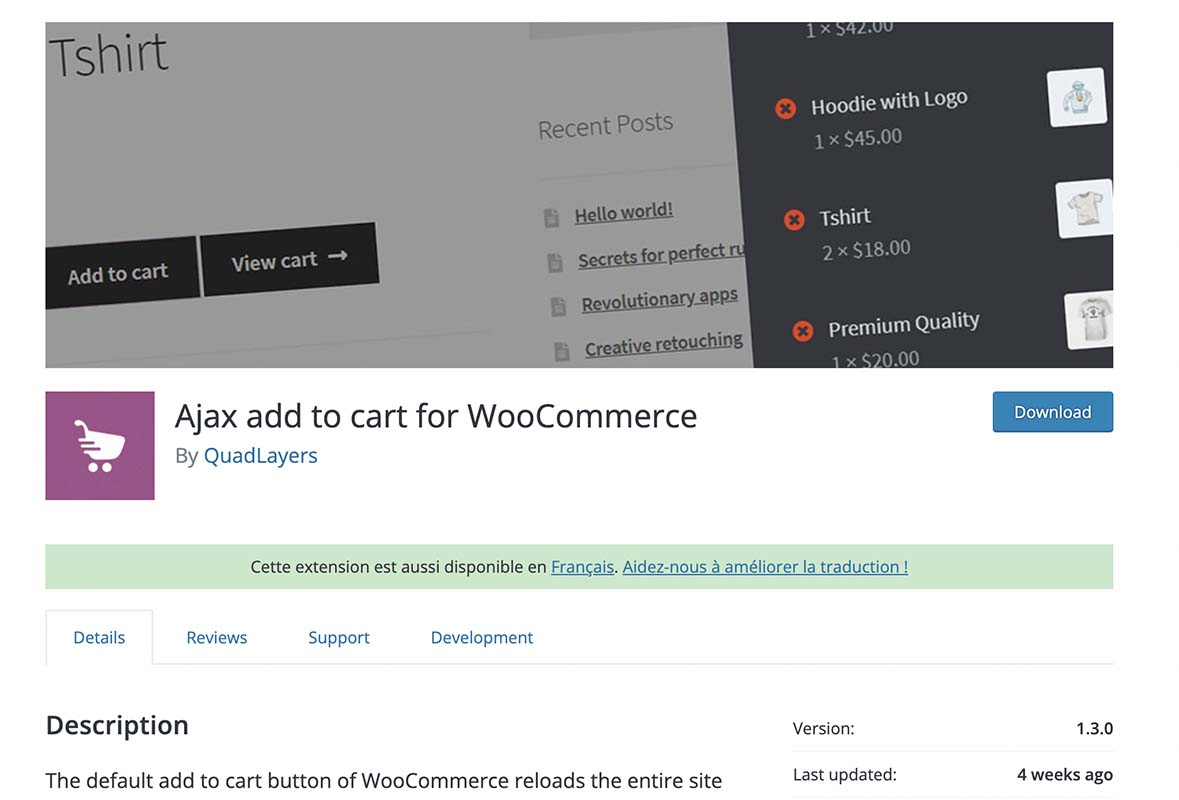
Ajax add to cart on WooCommerce
For developers:
Create a Child Theme by using Child Theme Generator.
A child theme is a theme that is created and inherits all the features and functions of the parent theme.
The main factor for creating it is that if you directly modify the theme’s files, the new files will override your changes, and all of your changes will be lost the next time the theme is updated. However, if you modify the child theme, the changes will not be overwritten.
Add the Javascript
Add the following code to the functions.php file in your child theme.
function woocommerce_ajax_add_to_cart_js() {
|
Create a new folder in your child’s theme called “JS” and add a new javascript called “ajax-add-to-cart.js” after adding the javascript.
Add jQuery to avoid recharging
Use this code to prevent information is sent to the database and reload the whole page.
(function ($) {
After that, you need to add the below code
|
It will step-by-step perform the process such as:
- Add the product to the cart
jQuery will be used to collect data from the website and add a product from the site to the cart. Incorporate this code into the previous code that hooks into the add to cart single product button event to collect product data.
- Use add to cart event
Following this code, in which we define an array with all required variables, we will fire the add to cart event, just in case a theme is using this function to make changes.
- Use add to cart function
- Use add to cart on single products script
Add to cart PHP function
Last but not least, the PHP function will be required. The simple way to implement your add to cart feature is to use some of the WooCommerce filter hooks. You will do it again this time, but with AJAX.
Let the script just below the previous script we used to add the Javascript file to the child theme.
add_action('wp_ajax_woocommerce_ajax_add_to_cart', 'woocommerce_ajax_add_to_cart');
|
Test the code
If you press “Enter” and it runs, so Congratulations!
If not, you should check the coding again.
Enable the “add to cart” button
You have to go to Dashboard > WooCommerce > Setting > Products tab.
NOTE:
What is the role of jQuery in “Ajax add to cart”?
The jQuery code was used to prevent information from being exchanged with the database and to reload the entire page. Using jQuery will only require a certain reload of the web page.
What are the Advantages and Disadvantages of “Ajax add to cart”?
- Advantage:
Improved User Experience: The most significant advantage is the rich user experience provided by AJAX.
By exchanging small amounts of data with the server, AJAX allows websites to update the serial number. This allows you to update parts of the site without having to reload the entire page. AJAX improves browser performance and allows for faster browsing speed, resulting in a more responsive user experience.
Enhanced User Productivity: The AJAX library includes object-oriented helper functions that boost productivity while decreasing frustration.
Reduce bandwidth usage and increase speed: AJAX communicates with the webserver and exchanges data using JavaScript via client-side scripting. Response times are faster when Ajax is used, so performance and speed are improved.
- Disadvantages:
Increase the load on the webserver: If you add a type of automatic update to the server every few seconds, the load may increase depending on the user.
Browser incompatibility: AJAX is heavily reliant on JavaScript, which is implemented differently in different browsers. This proves to be a drawback, especially when AJAX is required to work across browsers.
Wrapping up
In conclusion, the best way to set up an add to cart button on WooCommerce is by using the WooCommerce Ajax Cart plugin.
This plugin is highly customizable and easy to use. It’s a great option for those who want to add an “Ajax Add to Cart” button on their site that can update the total amount of their customers’ carts in the cart without having to refresh the page.